Learning Circuitry Part 3: The Non-Techie’s Guide to Programming
Programming, developing, coding, call it whatever you want, but sometimes all that can come across too “geeky” for musicians.
Aren’t musicians or producers supposed to be carving out their creativity rather than getting down into stuff that’s better done by people with technical skills?
Well, the answer is: not exactly. If for one minute you stop thinking of C++, JavaScript, etc. as tech stuff and start thinking of them as avenues to enhanced music production skills, then you can exceed in an unparalleled manner.
Imagine having control over the roots of a plant, the direction they go into. You can specify every single thing you want to if you have knowledge of what I’m about to tell you.
Arduino Programming Language
The C programming language is the world’s most popular programming language.
It’s called the father of many languages since C++ and JavaScript take a lot of concepts from it and refine them.
C, C++ and JavaScript might be different when broken down into technical pros & cons, however one thing they all have in common is their syntax.
Syntax is basically the sentence or statement you write in the programming software or console. In fact, the basic C syntax gained such popularity that ultimately high level languages like the ones used in micro-controllers starter borrowing theirs from them.
Arduino language itself is a subset of C/C++ functions, all of which can be called through the Arduino Workspace. All standard C and C++ characteristics are supported by Arduino IDE and once you’ve written the code, it all gets wired up to a C/C++ compiler before heading off to your device.
JavaScript
Out of the very large pool of programming languages, one that particularly hooks out is JavaScript.
Why? In three words, it is easy. Often referred to as JS, the programming language has been around for almost 2 decades now and shows no signs of going away. It is a high level language, meaning that it goes through a layer of translation before the computer executes it. This also means that the statements are extremely easy to understand and write as well.
Its importance is of such great level that it is one of the three main languages that form the basis of the Internet! That’s true. HTML, CSS and the third, JavaScript are the cornerstones of human advancement and thus should be treated with respect. JavaScript can make it possible for you to manipulate your webpage in countless manners and even though it isn’t that much focused on storing data, it can do a great deal for you both within web browsers as well as in desktop applications.
JavaScript is a case sensitive language. This means that the functions Alert() and alert() aren’t the same thing.
Also, you’ll need to end each statement with a semicolon. But don’t worry even if you forget, the interpreter will do it for you at the the backend.
In summary: Javascript is a fantastic language to learn the basics of programming with ease.
Table of Contents
- Getting started with Codepen.io
- Your First JS script: Using alert()
- Using console.log()
- Using variables (var)
- Functions
- Arrays
- Return Functions
Getting started with Codepen.io
We will be using one of my favorite tools to learn Javascript. Of course, you can write Javascript in any text editor and be able to preview the result in your web browser, but CodePen makes this a lot easier for educational purposes.
Visit “codepen.io”
This is a website that simulates HTML, CSS, and Javascript, elegantly displaying the result in real time. This means you can both develop applications as well as see them working without refreshing your browser.
Of course, we won’t be worrying about HTML or CSS. We will only be talking about Javascript.
All you have to do is Click on “New Pen”.
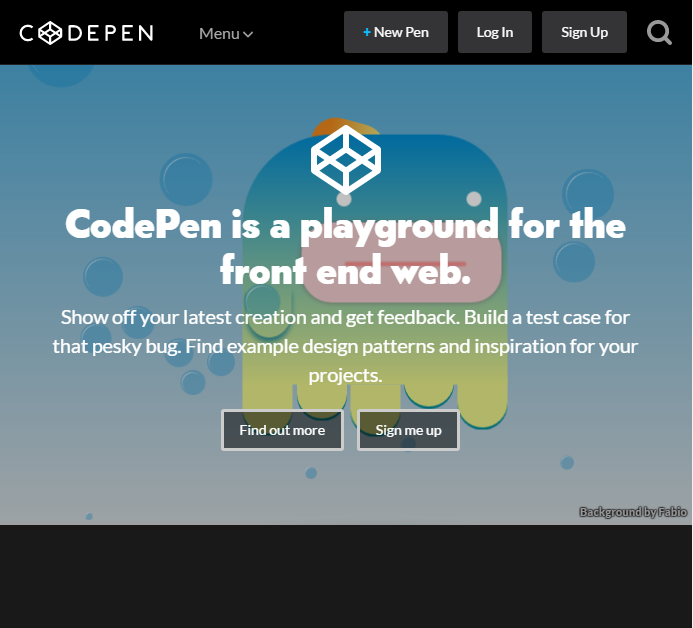
Next off, you’ll see three windows or boxes.
- HTML
- CSS
- JS
Ignore the first two as you’re here to learn JavaScript only.
Here’s how you can maximize the JavaScript window.
Your First JS script: Using alert()
As simple as it gets. Alert boxes are used to notify the users of any incoming information. You see them all the time. Here’s how to make one for yourself
In the “JS” box, simply write:
alert(“I am not a human being!”);
Using console.log()
The function, console.log(), is commonly used for debugging that comes in handy for web developers.
With this command you can get output in the web browser’s console.
Simply type in:
console.log(11 + 11);
Press on the Console button highlighted in blue in the screenshot below; you’ll have the answer.
Who would’ve guessed! 11+11 is equal to 22 and we were able to calculate that!
Using variables (var)
If you wish to carry out some kind of arithmetic operations using JavaScript, then you’ll have to first declare a block of memory for them.
How do you do that? By creating a “var”. The command simply reserves a memory chunk for a variable which can later be manipulated in any way you want.
Side note: In Arduino programming, the Javascript “var” is replaced with more specific indicators such as “int”, “float”, “String”, etc.
Not just arithmetic operations, you can carry out assignment, comparison and binary operations as well.
For now, arithmetic operations will do.
- Addition (+)
- Subtraction (-)
- Multiplication (*)
- Division (/)
- Modulus (%)
- Increment (++)
- Decrement (–)
Here’s an example piece of code:
var x = 6;
var y = 10;
var z = x + y;
console.log(z);
Boom! There are 3 variables. The vars “x” and “y’ are assigned to numbers and “z” is assigned to the sum of “x” and “y”.
In the same way you can carry out other operations as well or use the alert() command.
Functions
A function is basically a block of code, something that’s common in several programming languages. The block of code can be executed through a single line; the process is called “invoking”. A function can be called automatically, through the click of a button or from a code when the respective line is read.
function name(parameter1, parameter2, parameter3) { // This is where you would enter your code }
Over here, parameter 1, 2 and 3 are three variables that are to manipulated in some manner within the function. The user will provide them when he/she calls the function. The “name” can be anything.
Try this code:
function GameofThrones(parameter1, parameter2, parameter3) { var b = parameter1 + parameter2 + parameter3; alert(b); } GameofThrones(1,2,3);
Arrays
When storing a set of variables that are relatable, its best to go with Arrays. Think of them as a cupboard with drawers. The number of drawers is up to you!
Try the following code:
var names = [“John”, “Bill”, “Michael”];
alert(names);
Return Functions
If you want your function to give something back to the line of code from where it was called, then a Return Function is employed. The structure of the function is the same, with the addition of a single line of code.
function GameofThrones(parameter1, parameter2, parameter3) { return b; } var c = GameofThrones(1,2,3); alert(c);
Conclusion
Hope you enjoyed this article! JavaScript is a really simple, easy to understand programming language so no need to complicate it. More, coming up soon.
If you’ve already purchased the DIY MIDI Controller Bible, make sure to review some of the supplied code. It should make a lot more sense now!
Leave a Comment
4 comments
I could not refrain from commenting. Very well written!
Thanks!
Another in a series of excellent tutorials; I will direct interested students to your site and book.
Harold, I appreciate that greatly! Thanks for reading!